FastAPI-MCP is a zero-configuration device that seamlessly exposes FastAPI endpoints as Mannequin Context Protocol (MCP) instruments. It permits you to mount an MCP server immediately inside your FastAPI app, making integration easy.
On this tutorial, we’ll discover methods to use FastAPI-MCP by changing a FastAPI endpoint—which fetches alerts for U.S. nationwide parks utilizing the Nationwide Park Service API—into an MCP-compatible server. We’ll be working in Cursor IDE to stroll via this setup step-by-step.
Nationwide Park Service API
To make use of the Nationwide Park Service API, you possibly can request an API key by visiting this link and filling out a brief type. As soon as submitted, the API key will likely be despatched to your e mail.
Be certain to maintain this key accessible—we’ll be utilizing it shortly.
Cursor IDE Set up
You may obtain the Cursor IDE from cursor.com. It’s constructed particularly for AI-assisted growth. It’s free to obtain and comes with a 14-day free trial.
Python Dependencies
Run the next command to obtain the required libraries:
pip set up fastapi uvicorn httpx python-dotenv pydantic fastapi-mcp mcp-proxy
We will likely be making a easy FastAPI app that makes use of the Nationwide Park Service API to provide alerts associated to US Nationwide Parks. Later we are going to convert this app into an MCP server.
First create a .env file and retailer your API key
Exchange
from fastapi import FastAPI, HTTPException, Question
from typing import Listing, Elective
import httpx
import os
from dotenv import load_dotenv
from fastapi_mcp import FastApiMCP
# Load setting variables from .env file
load_dotenv()
app = FastAPI(title="Nationwide Park Alerts API")
# Get API key from setting variable
NPS_API_KEY = os.getenv("NPS_API_KEY")
if not NPS_API_KEY:
elevate ValueError("NPS_API_KEY setting variable shouldn't be set")
@app.get("/alerts")
async def get_alerts(
parkCode: Elective[str] = Question(None, description="Park code (e.g., 'yell' for Yellowstone)"),
stateCode: Elective[str] = Question(None, description="State code (e.g., 'wy' for Wyoming)"),
q: Elective[str] = Question(None, description="Search time period")
):
"""
Retrieve park alerts from the Nationwide Park Service API
"""
url = "https://developer.nps.gov/api/v1/alerts"
params = {
"api_key": NPS_API_KEY
}
# Add elective parameters if offered
if parkCode:
params["parkCode"] = parkCode
if stateCode:
params["stateCode"] = stateCode
if q:
params["q"] = q
attempt:
async with httpx.AsyncClient() as shopper:
response = await shopper.get(url, params=params)
response.raise_for_status()
return response.json()
besides httpx.HTTPStatusError as e:
elevate HTTPException(
status_code=e.response.status_code,
element=f"NPS API error: {e.response.textual content}"
)
besides Exception as e:
elevate HTTPException(
status_code=500,
element=f"Inner server error: {str(e)}"
)
if __name__ == "__main__":
import uvicorn
uvicorn.run(app, host="0.0.0.0", port=8000)
To check the app, run the next command within the terminal:
As soon as the server is working, open your browser and go to: http://localhost:8000/docs. It will open an interface the place we are able to take a look at our API endpoint
- Click on on the “Strive it out” button.
- Within the park_code parameter area, enter “ca” (for California parks).
- Click on “Execute”.
It’s best to obtain a 200 OK response together with a JSON payload containing alert data for nationwide parks in California.
To do that, add the next code simply earlier than the if __name__ == “__main__”: block in your app.py file:
mcp = FastApiMCP(
app,
# Elective parameters
title="Nationwide Park Alerts API",
description="API for retrieving alerts from Nationwide Parks",
base_url="http://localhost:8000",
)
mcp.mount()
.
Alternatively, you possibly can copy the next code and change your app.py with the identical:
from fastapi import FastAPI, HTTPException, Question
from typing import Listing, Elective
import httpx
import os
from dotenv import load_dotenv
from fastapi_mcp import FastApiMCP
# Load setting variables from .env file
load_dotenv()
app = FastAPI(title="Nationwide Park Alerts API")
# Get API key from setting variable
NPS_API_KEY = os.getenv("NPS_API_KEY")
if not NPS_API_KEY:
elevate ValueError("NPS_API_KEY setting variable shouldn't be set")
@app.get("/alerts")
async def get_alerts(
parkCode: Elective[str] = Question(None, description="Park code (e.g., 'yell' for Yellowstone)"),
stateCode: Elective[str] = Question(None, description="State code (e.g., 'wy' for Wyoming)"),
q: Elective[str] = Question(None, description="Search time period")
):
"""
Retrieve park alerts from the Nationwide Park Service API
"""
url = "https://developer.nps.gov/api/v1/alerts"
params = {
"api_key": NPS_API_KEY
}
# Add elective parameters if offered
if parkCode:
params["parkCode"] = parkCode
if stateCode:
params["stateCode"] = stateCode
if q:
params["q"] = q
attempt:
async with httpx.AsyncClient() as shopper:
response = await shopper.get(url, params=params)
response.raise_for_status()
return response.json()
besides httpx.HTTPStatusError as e:
elevate HTTPException(
status_code=e.response.status_code,
element=f"NPS API error: {e.response.textual content}"
)
besides Exception as e:
elevate HTTPException(
status_code=500,
element=f"Inner server error: {str(e)}"
)
mcp = FastApiMCP(
app,
# Elective parameters
title="Nationwide Park Alerts API",
description="API for retrieving alerts from Nationwide Parks",
base_url="http://localhost:8000",
)
mcp.mount()
if __name__ == "__main__":
import uvicorn
uvicorn.run(app, host="0.0.0.0", port=8000)
Subsequent, you’ll have to register your FastAPI MCP server in Cursor.
- Open Cursor and navigate to:
File > Preferences > Cursor Settings > MCP > Add a brand new international MCP server - It will open the mcp.json configuration file.
- Inside that file, add the next entry and reserve it:
{
"mcpServers": {
"Nationwide Park Service": {
"command": "mcp-proxy",
"args": ["http://127.0.0.1:8000/mcp"]
}
}
}
Now run the app utilizing the next command:
As soon as the app is working, navigate to File > Preferences > Cursor Settings > MCP. It’s best to now see your newly added server listed and working below the MCP part.
Now you can take a look at the server by getting into a immediate within the chat. It can use our MCP server to fetch and return the suitable end result.
Additionally, don’t overlook to comply with us on Twitter and be part of our Telegram Channel and LinkedIn Group. Don’t Overlook to affix our 90k+ ML SubReddit.
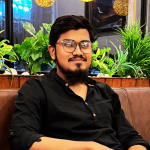
I’m a Civil Engineering Graduate (2022) from Jamia Millia Islamia, New Delhi, and I’ve a eager curiosity in Knowledge Science, particularly Neural Networks and their utility in numerous areas.